LangChain vs LlamaIndex: Choosing the Right Framework for Your LLM Application
Introduction:Large Language Models (LLMs) are now widely available for basic chatbot based usage, but integrating them into more complex applications can be difficult. Lucky for developers, there are tools that streamline the integration of LLMs to applications, two of the most prominent being LangChain and LlamaIndex. These two
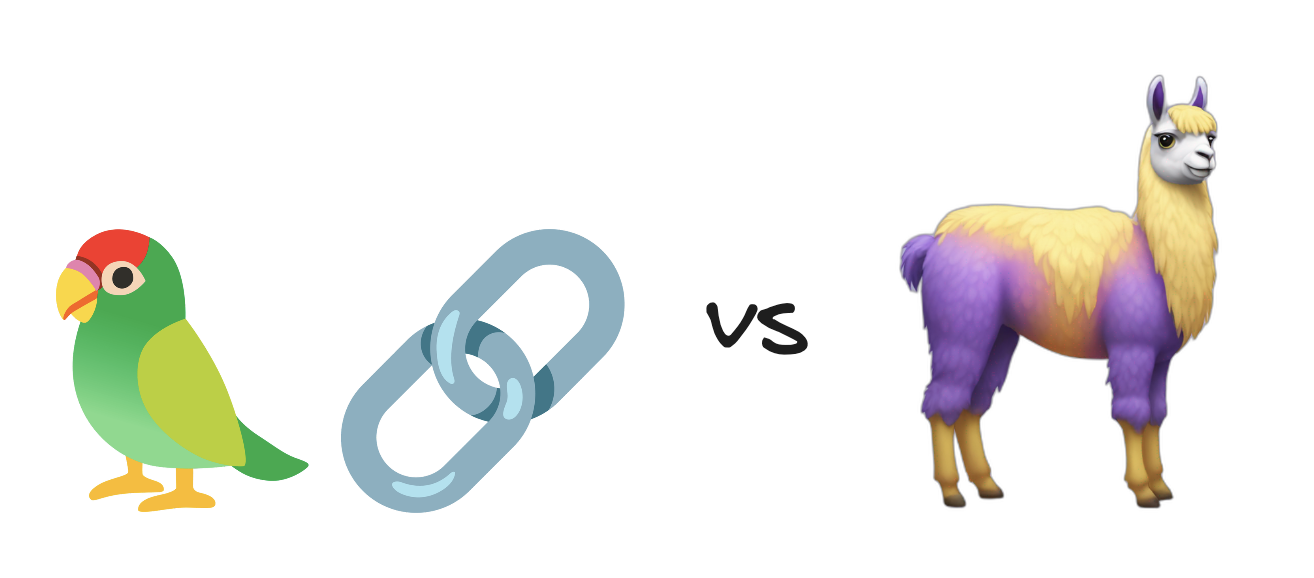
Introduction:
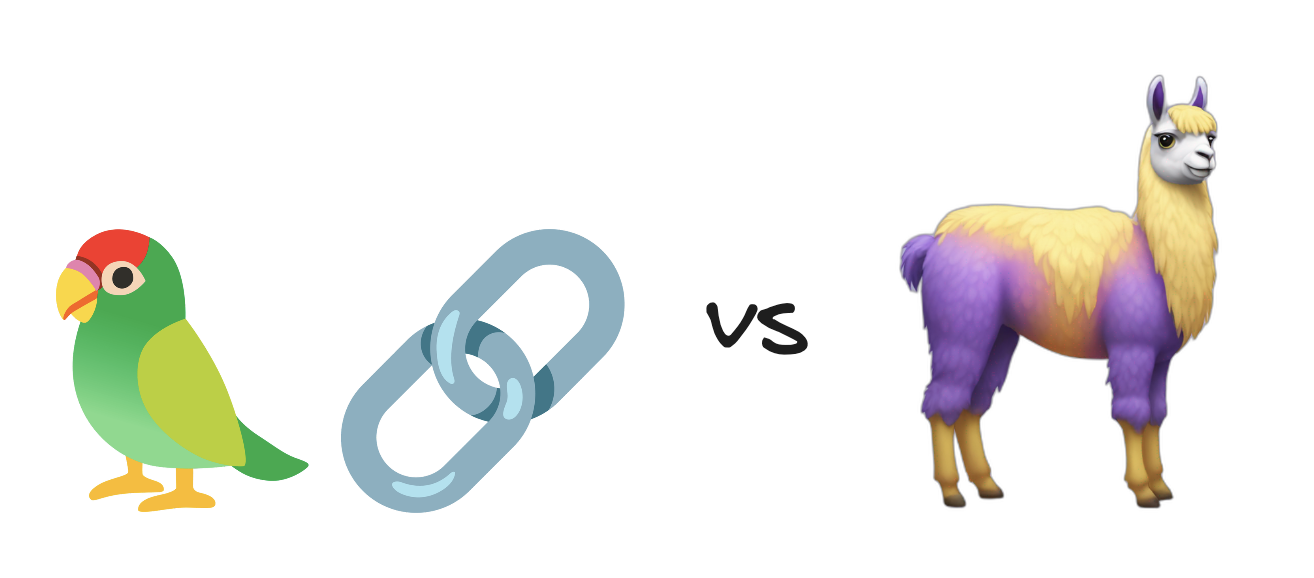
Large Language Models (LLMs) are now widely available for basic chatbot based usage, but integrating them into more complex applications can be difficult. Lucky for developers, there are tools that streamline the integration of LLMs to applications, two of the most prominent being LangChain and LlamaIndex.
These two open-source frameworks bridge the gap between the raw power of LLMs and practical, user-ready apps - each offering a unique set of tools supporting developers in their work with LLMs. These frameworks streamline key capabilities for developers, such as RAG workflows, data connectors, retrieval, and querying methods.
In this article, we will explore the purposes, features, and strengths of LangChain and LlamaIndex, providing guidance on when each framework excels. Understanding the differences will help you make the right choice for your LLM-powered applications.
Overview of Each Framework:
LangChain
Core Purpose & Philosophy:
LangChain was created to simplify the development of applications that rely on large language models by providing abstractions and tools to build complex chains of operations that can leverage LLMs effectively. Its philosophy centers around building flexible, reusable components that make it easy for developers to create intricate LLM applications without needing to code every interaction from scratch. LangChain is particularly suited to applications requiring conversation, sequential logic, or complex task flows that need context-aware reasoning.
Architecture
LangChain’s architecture is modular, with each component built to work independently or together as part of a larger workflow. This modular approach makes it easy to customize and scale, depending on the needs of the application. At its core, LangChain leverages chains, agents, and memory to provide a flexible structure that can handle anything from simple Q&A systems to complex, multi-step processes.
Key Features
Document loaders in LangChain are pre-built loaders that provide a unified interface to load and process documents from different sources and formats including PDFs, HTML, txt, docx, csv, etc. For example, you can easily load a PDF document using the PyPDFLoader, scrape web content using the WebBaseLoader, or connect to cloud storage services like S3. This functionality is particularly useful when building applications that need to process multiple data sources, such as document Q&A systems or knowledge bases.
from langchain.document_loaders import PyPDFLoader, WebBaseLoader
# Loading a PDF
pdf_loader = PyPDFLoader("document.pdf")
pdf_docs = pdf_loader.load()
# Loading web content
web_loader = WebBaseLoader("https://nanonets.com")
web_docs = web_loader.load()
Text splitters handle the chunking of documents into manageable contextually aligned pieces. This is a key precursor to accurate RAG pipelines. LangChain provides various splitting strategies for example the RecursiveCharacterTextSplitter, which splits text while attempting to maintain inter-chunk context and semantic meaning. You can configure chunk sizes and overlap to balance between context preservation and token limits.
from langchain.text_splitter import RecursiveCharacterTextSplitter
splitter = RecursiveCharacterTextSplitter(
chunk_size=1000,
chunk_overlap=200,
separators=["\n\n", "\n", " ", ""]
)
chunks = splitter.split_documents(documents)
Prompt templates aid in standardizing prompts for various tasks, ensuring consistency across interactions. LangChain allows you to define these reusable templates with variables that can be filled dynamically, which is a powerful feature for creating consistent but customizable prompts. This consistency means your application will be easier to maintain and update when necessary. A good technique to employ within your templates is ‘few-shot’ prompting, in other words, including examples (positive and negative).
from langchain.prompts import PromptTemplate
# Define a few-shot template with positive and negative examples
template = PromptTemplate(
input_variables=["topic", "context"],
template="""Write a summary about {topic} considering this context: {context}
Examples:
### Positive Example 1:
Topic: Climate Change
Context: Recent research on the impacts of climate change on polar ice caps
Summary: Recent studies show that polar ice caps are melting at an accelerated rate due to rising global temperatures. This melting contributes to rising sea levels and impacts ecosystems reliant on ice habitats.
### Positive Example 2:
Topic: Renewable Energy
Context: Advances in solar panel efficiency
Summary: Innovations in solar technology have led to more efficient panels, making solar energy a more viable and cost-effective alternative to fossil fuels.
### Negative Example 1:
Topic: Climate Change
Context: Impacts of climate change on polar ice caps
Summary: Climate change is happening everywhere and has effects on everything. (This summary is vague and lacks detail specific to polar ice caps.)
### Negative Example 2:
Topic: Renewable Energy
Context: Advances in solar panel efficiency
Summary: Renewable energy is good because it helps the environment. (This summary is overly general and misses specifics about solar panel efficiency.)
### Now, based on the topic and context provided, generate a detailed, specific summary:
Topic: {topic}
Context: {context}
Summary:"""
)
# Format the prompt with a new example
prompt = template.format(topic="AI", context="Recent developments in machine learning")
print(prompt)
LCEL represents the modern approach to building chains in LangChain, offering a declarative way to compose LangChain components. It's designed for production-ready applications from the start, supporting everything from simple prompt-LLM combinations to complex multi-step chains. LCEL provides built-in streaming support for optimal time-to-first-token, automatic parallel execution of independent steps, and comprehensive tracing through LangSmith. This makes it particularly valuable for production deployments where performance, reliability, and observability are necessary. For example, you could build a retrieval-augmented generation (RAG) pipeline that streams results as they're processed, handles retries automatically, and provides detailed logging of each step.
from langchain.chat_models import ChatOpenAI
from langchain.prompts import ChatPromptTemplate
from langchain.schema.output_parser import StrOutputParser
# Simple LCEL chain
prompt = ChatPromptTemplate.from_messages([
("system", "You are a helpful assistant."),
("user", "{input}")
])
chain = prompt | ChatOpenAI() | StrOutputParser()
# Stream the results
for chunk in chain.stream({"input": "Tell me a story"}):
print(chunk, end="", flush=True)
Chains are one of LangChain's most powerful features, allowing developers to create sophisticated workflows by combining multiple operations. A chain might start with loading a document, then summarizing it, and finally answering questions about it. Chains are primarily created using LCEL (LangChain Execution Language). This tool makes it straightforward to both construct custom chains and use ready-made, off-the-shelf chains.
There are several prebuilt LCEL chains available:
- create_stuff_document_chain: Use when you want to format a list of documents into a single prompt for the LLM. Ensure it fits within the LLM’s context window as all documents are included.
- load_query_constructor_runnable: Generates queries by converting natural language into allowed operations. Specify a list of operations before using this chain.
- create_retrieval_chain: Passes a user inquiry to a retriever to fetch relevant documents. These documents and the original input are then used by the LLM to generate a response.
- create_history_aware_retriever: Takes in conversation history and uses it to generate a query, which is then passed to a retriever.
- create_sql_query_chain: Suitable for generating SQL database queries from natural language.
Legacy Chains: There are also several chains available from before LCEL was developed. For example, SimpleSequentialChain, and LLMChain.
from langchain.chains import SimpleSequentialChain, LLMChain
from langchain.llms import OpenAI
import os
os.environ['OPENAI_API_KEY'] = "YOUR_API_KEY"
llm=OpenAI(temperature=0)
summarize_chain = LLMChain(llm=llm, prompt=summarize_template)
categorize_chain = LLMChain(llm=llm, prompt=categorize_template)
full_chain = SimpleSequentialChain(
chains=[summarize_chain, categorize_chain],
verbose=True
)
Agents represent a more autonomous approach to task completion in LangChain. They can make decisions about which tools to use based on user input and can execute multi-step plans to achieve goals. Agents can access various tools like search engines, calculators, or custom APIs, and they can decide how to use these tools in response to user requests. For instance, an agent might help with research by searching the web, summarizing findings, and formatting the results. LangChain has several types of agents including Tool Calling, OpenAI Tools/Functions, Structured Chat, JSON Chat, ReAct, and Self Ask with Search.
from langchain.agents import create_react_agent, Tool
from langchain.tools import DuckDuckGoSearchRun
search = DuckDuckGoSearchRun()
tools = [
Tool(
name="Search",
func=search.run,
description="useful for searching information online"
)
]
agent = create_react_agent(tools, llm, prompt)
Memory systems in LangChain enable applications to maintain context across interactions. This enables the creation of coherent conversational experiences or maintaining of state in long-running processes. LangChain offers various memory types, from simple conversation buffers to more sophisticated trimming and summary-based memory systems. For example, you could use conversation memory to maintain context in a customer service chatbot, or entity memory to track specific details about users or topics over time.
There are different types of memory in LangChain, depending on the level of retention and complexity:
- Basic Memory Setup: For a basic memory approach, messages are passed directly into the model prompt. This simple form of memory uses the latest conversation history as context for responses, allowing the model to answer with reference to recent exchanges. ‘conversationbuffermemory’ is a good example of this.
- Summarized Memory: For more complex scenarios, summarized memory distills previous conversations into concise summaries. This approach can improve performance by replacing verbose history with a single summary message, which maintains essential context without overwhelming the model. A summary message is generated by prompting the model to condense the full chat history, which can then be updated as new interactions occur.
- Automatic Memory Management with LangGraph: LangChain’s LangGraph enables automatic memory persistence by using checkpoints to manage message history. This method allows developers to build chat applications that automatically remember conversations over long sessions. Using the MemorySaver checkpointer, LangGraph applications can maintain a structured memory without external intervention.
- Message Trimming: To manage memory efficiently, especially when dealing with limited model context, LangChain offers the trim_messages utility. This utility allows developers to keep only the most recent interactions by removing older messages, thereby focusing the chatbot on the latest context without overloading it.
from langchain.memory import ConversationBufferMemory
from langchain.chains import ConversationChain
memory = ConversationBufferMemory()
conversation = ConversationChain(
llm=llm,
memory=memory,
verbose=True
)
# Memory maintains context across interactions
conversation.predict(input="Hi, I'm John")
conversation.predict(input="What's my name?") # Will remember "John"
LangChain is a highly modular, flexible framework that simplifies building applications powered by large language models through well-structured components. With its many features—document loaders, customizable prompt templates, and advanced memory management—LangChain allows developers to handle complex workflows efficiently. This makes LangChain ideal for applications that require nuanced control over interactions, task flows, or conversational state. Next, we'll examine LlamaIndex to see how it compares!
LlamaIndex
Core Purpose & Philosophy:
LlamaIndex is a framework designed specifically for efficient data indexing, retrieval, and querying to enhance interactions with large language models. Its core purpose is to connect LLMs with unstructured data, making it easy for applications to retrieve relevant information from massive datasets. The philosophy behind LlamaIndex is centered around creating flexible, scalable data indexing solutions that allow LLMs to access relevant data on-demand, which is particularly beneficial for applications focused on document retrieval, search, and Q&A systems.
Architecture
LlamaIndex’s architecture is optimized for retrieval-heavy applications, with an emphasis on data indexing, flexible querying, and efficient memory management. Its architecture includes Nodes, Retrievers, and Query Engines, each designed to handle specific aspects of data processing. Nodes handle data ingestion and structuring, retrievers facilitate data extraction, and query engines streamline querying workflows, all of which work in tandem to provide fast and reliable access to stored data. LlamaIndex’s architecture enables it to connect seamlessly with vector databases, enabling scalable and high-speed document retrieval.
Key Features
Documents and Nodes are data storage and structuring units in LlamaIndex that break down large datasets into smaller, manageable components. Nodes allow data to be indexed for rapid retrieval, with customizable chunking strategies for various document types (e.g., PDFs, HTML, or CSV files). Each Node also holds metadata, making it possible to filter and prioritize data based on context. For example, a Node might store a chapter of a document along with its title, author, and topic, which helps LLMs query with higher relevance.
from llama_index.core.schema import TextNode, Document
from llama_index.core.node_parser import SimpleNodeParser
# Create nodes manually
text_node = TextNode(
text="LlamaIndex is a data framework for LLM applications.",
metadata={"source": "documentation", "topic": "introduction"}
)
# Create nodes from documents
parser = SimpleNodeParser.from_defaults()
documents = [
Document(text="Chapter 1: Introduction to LLMs"),
Document(text="Chapter 2: Working with Data")
]
nodes = parser.get_nodes_from_documents(documents)
Retrievers are responsible for querying the indexed data and returning relevant documents to the LLM. LlamaIndex provides various retrieval methods, including traditional keyword-based search, dense vector-based retrieval for semantic search, and hybrid retrieval that combines both. This flexibility allows developers to select or combine retrieval techniques based on their application’s needs. Retrievers can be integrated with vector databases like FAISS or KDB.AI for high-performance, large-scale search capabilities.
from llama_index.core import VectorStoreIndex, SimpleDirectoryReader
from llama_index.core.retrievers import VectorIndexRetriever
# Create an index
documents = SimpleDirectoryReader('.').load_data()
index = VectorStoreIndex.from_documents(documents)
# Vector retriever
vector_retriever = VectorIndexRetriever(
index=index,
similarity_top_k=2
)
# Retrieve nodes
query = "What is LlamaIndex?"
vector_nodes = vector_retriever.retrieve(query)
print(f"Vector Results: {[node.text for node in vector_nodes]}")
Query Engines act as the interface between the application and the indexed data, handling and optimizing search queries to deliver the most relevant results. They support advanced querying options such as keyword search, semantic similarity search, and custom filters, allowing developers to create sophisticated, contextualized search experiences. Query engines are adaptable, supporting parameter tuning to refine search accuracy and relevance, and making it possible to integrate LLM-driven applications directly with data sources.
from llama_index.core import VectorStoreIndex, SimpleDirectoryReader, Settings
from llama_index.llms.openai import OpenAI
from llama_index.core.node_parser import SentenceSplitter
import os
os.environ['OPENAI_API_KEY'] = "YOUR_API_KEY"
GENERATION_MODEL = 'gpt-4o-mini'
llm = OpenAI(model=GENERATION_MODEL)
Settings.llm = llm
# Create an index
documents = SimpleDirectoryReader('.').load_data()
index = VectorStoreIndex.from_documents(documents, transformations=[SentenceSplitter(chunk_size=2048, chunk_overlap=0)],)
query_engine = index.as_query_engine()
response = query_engine.query("What is LlamaIndex?")
print(response)
LlamaIndex offers data connectors that allow for seamless ingestion from diverse data sources, including databases, file systems, and cloud storage. Connectors handle data extraction, processing, and chunking, enabling applications to work with large, complex datasets without manual formatting. This is especially helpful for applications requiring multi-source data fusion, like knowledge bases or extensive document repositories.
Other specialized data connectors are available on LlamaHub, a centralized repository within the LlamaIndex framework. These are prebuilt connectors within a unified and consistent interface that developers can use to integrate and pull in data from various sources. By using LlamaHub, developers can quickly set up data pipelines that connect their applications to external data sources without needing to build custom integrations from scratch.
LlamaHub is also open-source, so it is open to community contributions and new connectors and improvements are frequently added.
LlamaIndex allows for the creation of advanced indexing structures, such as vector indexes, and hierarchical or graph-based indexes, to suit different types of data and queries. Vector indexes enable semantic similarity search, hierarchical indexes allow for organized, tree-like layered indexing, while graph indexes capture relationships between documents or sections, enhancing retrieval for complex, interconnected datasets. These indexing options are ideal for applications that need to retrieve highly specific information or navigate complex datasets, such as research databases or document-heavy workflows.
from llama_index.core import VectorStoreIndex, SimpleDirectoryReader
# Load documents and build index
documents = SimpleDirectoryReader("../../path_to_directory").load_data()
index = VectorStoreIndex.from_documents(documents)
With LlamaIndex, data can be filtered based on metadata, like tags, timestamps, or other contextual information. This filtering enables precise retrieval, especially in cases where data segmentation is needed, such as filtering results by category, recency, or relevance.
from llama_index.core import VectorStoreIndex, Document
from llama_index.core.retrievers import VectorIndexRetriever
from llama_index.core.vector_stores import MetadataFilters, ExactMatchFilter
# Create documents with metadata
doc1 = Document(text="LlamaIndex introduction.", metadata={"topic": "introduction", "date": "2024-01-01"})
doc2 = Document(text="Advanced indexing techniques.", metadata={"topic": "indexing", "date": "2024-01-05"})
doc3 = Document(text="Using metadata filtering.", metadata={"topic": "metadata", "date": "2024-01-10"})
# Create and build an index with documents
index = VectorStoreIndex.from_documents([doc1, doc2, doc3])
# Define metadata filters, filter on the ‘date’ metadata column
filters = MetadataFilters(filters=[ExactMatchFilter(key="date", value="2024-01-05")])
# Set up the vector retriever with the defined filters
vector_retriever = VectorIndexRetriever(index=index, filters=filters)
# Retrieve nodes
query = "efficient indexing"
vector_nodes = vector_retriever.retrieve(query)
print(f"Vector Results: {[node.text for node in vector_nodes]}")
>>> Vector Results: ['Advanced indexing techniques.']
See another metadata filtering example here.
When to Choose Each Framework
LangChain Primary Focus
Complex Multi-Step Workflows
LangChain's core strength lies in orchestrating sophisticated workflows that involve multiple interacting components. Modern LLM applications often require breaking down complex tasks into manageable steps that can be processed sequentially or in parallel. LangChain provides a robust framework for chaining operations while maintaining clear data flow and error handling, making it ideal for systems that need to gather, process, and synthesize information across multiple steps.
Key capabilities:
- LCEL for declarative workflow definition
- Built-in error handling and retry mechanisms
Extensive Agent Capabilities
The agent system in LangChain enables autonomous decision-making in LLM applications. Rather than following predetermined paths, agents dynamically choose from available tools and adapt their approach based on intermediate results. This makes LangChain particularly valuable for applications that need to handle unpredictable user requests or navigate complex decision trees, such as research assistants or advanced customer service systems.
Common agent tools:
- Web search
- API integrations (Dall-E, DuckDuckGoSearch, Gmail, etc..).
- Code Interpreters
Custom tool creation for specific domains and use-cases
Memory Management
LangChain's approach to memory management solves the challenge of maintaining context and state across interactions. The framework provides sophisticated memory systems that can track conversation history, maintain entity relationships, and store relevant context efficiently.
LlamaIndex Primary Focus
Advanced Data Retrieval
LlamaIndex excels in making large amounts of custom data accessible to LLMs efficiently. The framework provides sophisticated indexing and retrieval mechanisms that go beyond simple vector similarity searches, understanding the structure and relationships within your data. This becomes particularly valuable when dealing with large document collections or technical documentation that require precise retrieval. For example, in dealing with large libraries of financial documents, retrieving the right information is a must.
Key retrieval features:
- Multiple retrieval strategies (vector, keyword, hybrid)
- Customizable relevance scoring (measure if query was actually answered by the systems response)
RAG Applications
While LangChain is very capable for RAG pipelines, LlamaIndex also provides a comprehensive suite of tools specifically designed for Retrieval-Augmented Generation applications. The framework handles complex tasks of document processing, chunking, and retrieval optimization, allowing developers to focus on building applications rather than managing RAG implementation details.
RAG optimizations:
- Advanced chunking strategies
- Context window management
- Response synthesis techniques
- Reranking
Making the Choice
The decision between frameworks often depends on your application's primary complexity:
- Choose LangChain when your focus is on process orchestration, agent behavior, and complex workflows
- Choose LlamaIndex when your priority is data organization, retrieval, and RAG implementation
- Consider using both frameworks together for applications requiring both sophisticated workflows and advanced data handling
It is also important to remember, in many cases, either of these frameworks will be able to complete your task. They each have their strengths, but for basic use-cases such as a naive RAG workflow, either LangChain or LlamaIndex will do the job. In some cases, the main determining factor might be which framework you are most comfortable working with.
Can I Use Both Together?
Yes, you can indeed use both LangChain and LlamaIndex together. This combination of frameworks can provide a powerful foundation for building production-ready LLM applications that handle both process and data complexity effectively. By integrating the two frameworks, you can leverage the strengths of each and create sophisticated applications that seamlessly index, retrieve, and interact with extensive information in response to user queries.
An example of this integration could be wrapping LlamaIndex functionality like indexing or retrieval within a custom LangChain agent. This would capitalize on the indexing or retrieval strengths of LlamaIndex, with the orchestration and agentic strengths of LangChain.
Summary Table:
Aspect | LangChain | LlamaIndex |
---|---|---|
Core Purpose | Building complex LLM applications with focus on workflow orchestration and chains of operations | Specialized in data indexing, retrieval, and querying for LLM interactions |
Primary Strengths | - Multi-step workflows orchestration - Agent-based decision making - Sophisticated memory management - Complex task flows | - Advanced data retrieval - Structured data handling - RAG optimizations - Data indexing structures |
Key Features | - Document Loaders - Text Splitters - Prompt Templates - LCEL (LangChain Expression Language) - Chains - Agents - Memory Management Systems | - Documents & Nodes - Retrievers - Query Engines - Data Connectors - LlamaHub - Advanced Index Structures - Metadata Filtering |
Best Used For | - Applications requiring complex workflows - Systems needing autonomous decision-making - Projects with multi-step processes Conversational applications | - Large-scale data retrieval - Document search systems - RAG implementations - Knowledge bases - Technical documentation handling |
Architecture Focus | Modular components for building chains and workflows | Optimized for retrieval-heavy applications and data indexing |
Conclusion
Choosing between LangChain and LlamaIndex depends on aligning each framework's strengths with your application’s needs. LangChain excels at orchestrating complex workflows and agent behavior, making it ideal for dynamic, context-aware applications with multi-step processes. LlamaIndex, meanwhile, is optimized for data handling, indexing, and retrieval, perfect for applications requiring precise access to structured and unstructured data, such as RAG pipelines.
For process-driven workflows, LangChain is likely the best fit, while LlamaIndex is ideal for advanced data retrieval methods. Combining both frameworks can provide a powerful foundation for applications needing sophisticated workflows and robust data handling, streamlining development and enhancing AI solutions.